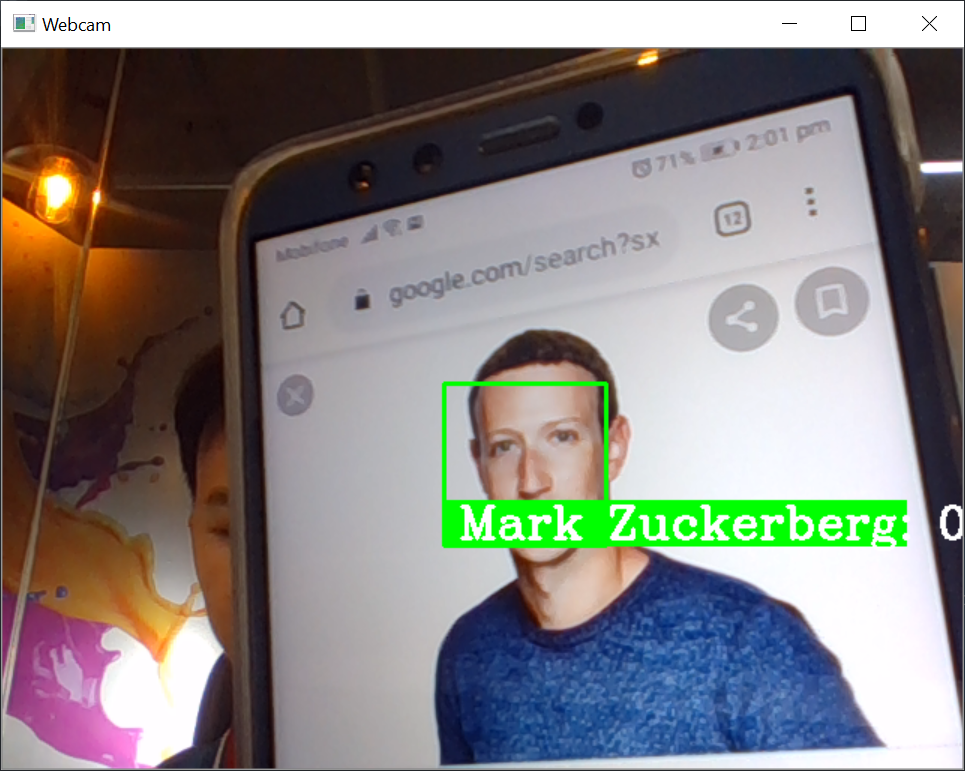
import cv2
import face_recognition
import numpy as np
import os
from datetime import datetime
pathImages = "ImagesAttendance"
myImgList = os.listdir(pathImages)
imagesObjKnown = []
nameList = []
for x, img in enumerate(myImgList):
imgCur = cv2.imread(f'{pathImages}/{img}')
imagesObjKnown.append(imgCur)
nameList.append(os.path.splitext(img)[0])
def findEncodeList(imageList):
encodeList = []
for image in imageList:
img = cv2.cvtColor(image, cv2.COLOR_BGR2RGB)
encode = face_recognition.face_encodings(img)[0]
encodeList.append(encode)
return encodeList
encodeListKnown = findEncodeList(imagesObjKnown)
print("Encode Complete")
def markAttendance(name):
with open('Attendance.csv', 'r+') as f:
now = datetime.now()
strTime = now.strftime('%d-%m-%Y %H:%M')
f.readlines()
f.writelines(f'\n{name}, {strTime}')
cap = cv2.VideoCapture(0)
while True:
success, imgWebcam = cap.read()
imgFrame = cv2.cvtColor(imgWebcam, cv2.COLOR_BGR2RGB)
imgFrameLoc = face_recognition.face_locations(imgFrame)
imgFrameEncode = face_recognition.face_encodings(imgFrame, imgFrameLoc)
for imgLoc, imgEncode in zip(imgFrameLoc, imgFrameEncode):
matches = face_recognition.compare_faces(encodeListKnown, imgEncode)
faceDis = face_recognition.face_distance(encodeListKnown, imgEncode)
matchIndex = np.argmin(faceDis)
if matches[matchIndex]:
name = nameList[matchIndex]
y1, x2, y2, x1 = imgLoc
cv2.rectangle(imgWebcam, (x1, y1), (x2, y2), (0, 255, 0), 2)
cv2.rectangle(imgWebcam, (x1, y2-30), (x2+200, y2), (0, 255, 0), cv2.FILLED)
cv2.putText(imgWebcam, f'{name}: {round(faceDis[matchIndex], 2)}', (x1+10, y2-5), cv2.FONT_HERSHEY_COMPLEX, 1, (255, 255, 255), 2)
markAttendance(name)
cv2.imshow("Webcam", imgWebcam)
if cv2.waitKey(1) & 0xFF == ord("q"):
break
Comments